Getting started with the DevExpress ASP.NET Office Controls is easy. In a few of the upcoming blogs, I'll highlight some of the common getting started tasks with DevExpress ASP.NET Rich Text Editor and ASP.NET Spreadsheet controls.
One of the first things you'll likely want to do after adding a DevExpress ASP.NET Office control to your web project is to open a document.
File System or Database
There are two ways to load a document into a DevExpress ASP.NET office control. You can use the file system or load it from document storage (database).
Note, the code example below reference the DevExpress ASP.NET Spreadsheet control, however, the same code applies to the DevExpress ASP.NET RichEdit control.
Load from File System
The DevExpress Office controls support various formats including docx, csv, pdf, xlsx, and more.
To load or open a document (docx, pdf, xlsx, etc.) using the Server-side API, call the Open method:
void ASPxSpreadsheet.Open(string pathToDocument)
The pathToDocument) parameter represents the full the document path and filename in the File System. The pathToDocument also plays role of the document identifier - the DocumentID.
The Open method also provides several overloads:
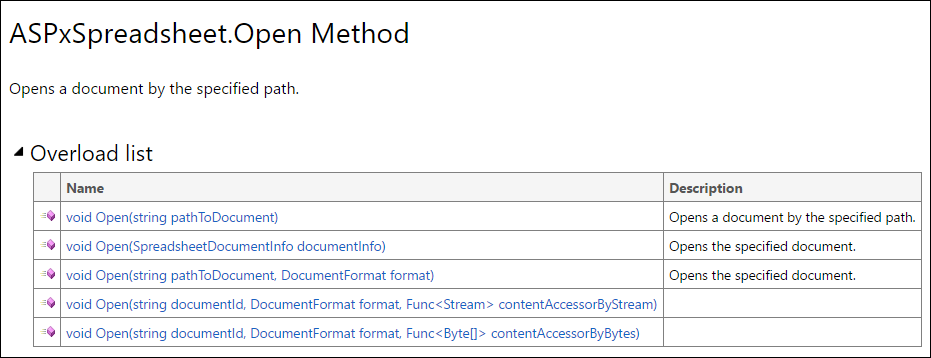
Because these are server-side ASP.NET controls, the file system mentioned above is the one on your web server. End-users can upload a file to the working directory that you specify for the control to use. Then, call the open method and load the corresponding file into the DevExpress ASP.NET Office control.
Take a look at this 'Custom Document Management' demo which has integrated the versatile DevExpress ASP.NET File Manager control. The File Manager control gives your end-users a visual layout of the server-side file system and allows them to upload files too.
Load from Document Storage (Database)
To load a document from the database, the DevExpress Office Controls provide stream and byte array parameters in the Open method:
void ASPxSpreadsheet.Open(string documentId, DocumentFormat format, Func<Stream> contentAccessor)
void ASPxSpreadsheet.Open(string documentId, DocumentFormat format, Func<byte[]> contentAccessor)
Let's take a look at the parameters needed to retrieve a file (aka binary object) from your database.
DocumentID
The DocumentID parameter is simply a unique string and is used to load and save documents. The DocumentID is necessary when dealing with ASP.NET documents programmatically.
If you've previously saved your DocumentID parameter to the database then you can use this ID for the open method.
Or you can also generate a new unique ID using the following approach:
private void CustomDocumentOpening() {
var newUniqueDocumentId = Guid.NewGuid().ToString();
DocumentFormat
The DocumentFormat specifies the file format you want to open (xlsx, docx, etc.).
Load Blob from Database
After getting your DocumentID, you'll need to write some custom code that extracts the document (binary object) from your database.
For example, here is how to retrieve it via a stream:
// Open document from content in stream
using(var stream = GetStreamFromCustomDocumentStorage()) {
ASPxSpreadsheet1.Open(newUniqueDocumentId, DocumentFormat.Xlsx, () => stream);
}
}
private FileStream GetStreamFromCustomDocumentStorage() {
throw new NotImplementedException();
}
And here is how to retrieve it via a byte array:
// Or, open document from content in byte array
byte[] documentContentAsByteArray = GetByteArrayFromCustomDocumentStorage();
ASPxSpreadsheet1.Open(newUniqueDocumentId, DocumentFormat.Xlsx, () => documentContentAsByteArray);
// TODO save somewhere the newUniqueDocumentId if needed
}
private byte[] GetByteArrayFromCustomDocumentStorage() {
throw new NotImplementedException();
}
Once the document is retrieved from the database, it can be passed to one of the open method overloads as a Stream or array of bytes (along with the DocumentID and DocumentFormat).
Feedback
I would love to hear your feedback on the DevExpress ASP.NET Office Controls.
Leave me a comment below or email me directly: mharry@devexpress.com
Thanks!
Your Next Great .NET App Starts Here
Year after year, .NET developers such as yourself consistently vote DevExpress products #1.
Experience the DevExpress difference for yourself and download a free 30-day trial of all our products today: DevExpress.com/trial.
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.