One of our ongoing priorities is to continuously refine existing features based on customer feedback. Your feedback allows us to devote more resources to what matters most, and helps us better understand a diverse range of possible usage scenarios.
In the case of Organize Members, many of you have asked for the ability to group members by name (or by the member’s type). In response, we’ve added this functionality (in v20.2.3 and up).
To group members, you can use the Contains, Starts With, and Equals string operations (along with RegEx expressions) on the member name or on the member’s type name (e.g., method return type or the type of property or variable).
Let’s explore a few scenarios where grouping by member type or member name can help organize your code, improving readability and clarity.
Example 1 - Grouping All Members of a Specific Type
When developing WPF applications with MVVM, it is common to have classes with ICommand properties, and it may make sense to organize this code so the ICommand properties are grouped together.
To get CodeRush to organize your ICommand properties, follow these steps:
Open the Organize Members options page and create a new “ICommand properties” rule, as shown in the screenshot below, to group properties (both auto-implemented and normal) by the ICommand type:
.png)
Click OK to save your changes and close the options page.
As an example, if we start with the following code:
using System;
using System.Windows.Input;
namespace WpfApp1.Folder
{
class CodePlacesMenuViewModel : ItemViewModelBase
{
bool autoHide;
private void SetProperty(ref T autoHide, T value, string v, object onAutoHideChanged)
{
throw new NotImplementedException();
}
public ICommand ExpandAllCommand { get; set; }
public bool AutoHide {
get => autoHide;
set => SetProperty(ref autoHide, value, nameof(AutoHide), OnAutoHideChanged);
}
public ICommand CollapseAllCommand { get; set; }
public object OnAutoHideChanged { get; private set; }
public ICommand AutoHideBtnCommand { get; set; }
}
}
Running Organize Members with this new rule would produce a result like this, grouping all your ICommand properties at the bottom of the class:

Example 2 - Grouping Event Handlers by Name
In the area of WinForms application development, it is not unusual for files to contain event handlers strewn throughout the code. And in some cases, we can make the code easier to read if we group and order event handlers in a way that makes sense.
As an example, we might want the Form’s Load event handler to follow the form’s constructor. This can keep our initialization code in the same place so it’s easier to understand what happens when we first present the form. This Form.Load event handler is typically named FormXxx_Load.
You can create a new “Form_Load” rule and use the Contains operation to only apply the rule if the member name contains the text “_Load”, as shown below. To change a rule’s position in the rule set, simply drag the rule and drop it where you need it (in our example, we would drop this rule right after the Constructors rule, and as a result, all methods containing the text “_Load” will be placed after the constructor).
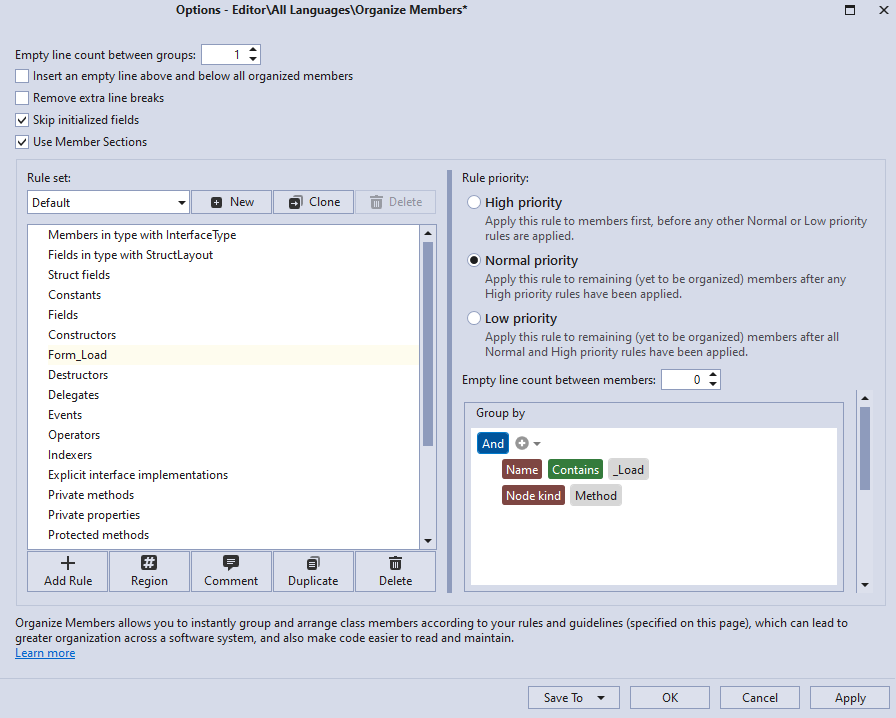
You can also use regular expressions to specify more complex conditions to match. For example, to group the event handler using the “*Form*_Load*” mask, you could specify the following regex matching constraint for the “Form_Load” rule:
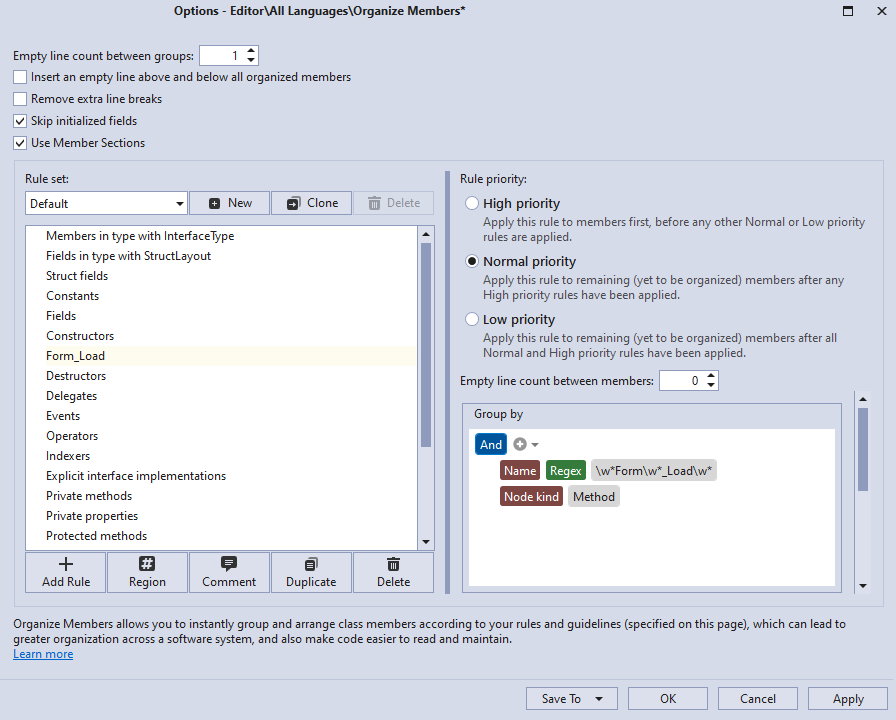
It can be also useful to group all handlers of a certain event for components that belong to one type (for example, Button.Click). Consider controls with the following names: button1, button2, etc., and specify the “Button click event handlers” rule:
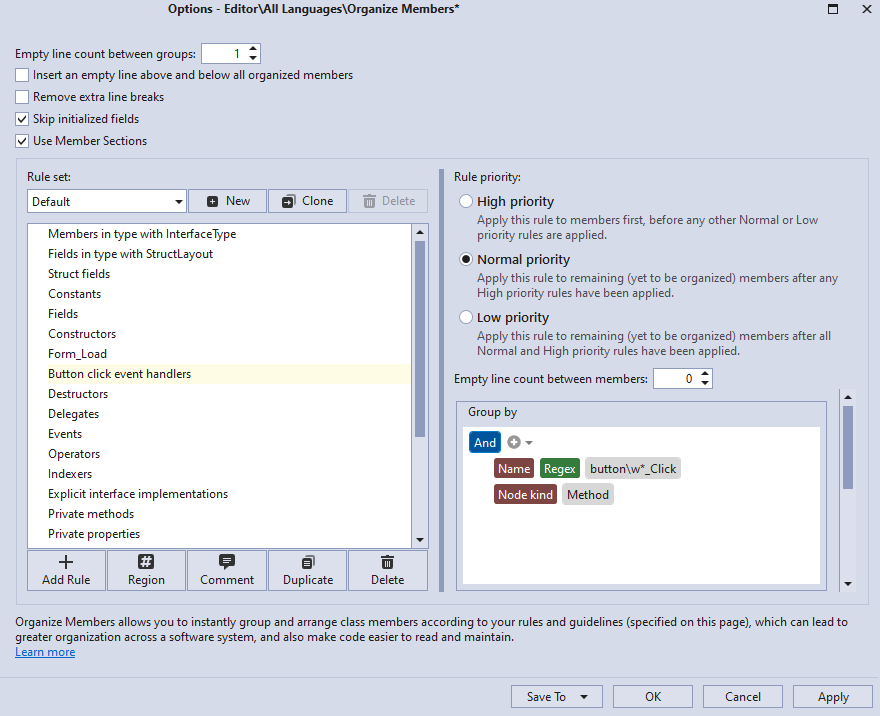
Click OK to save the changes and close the options page.
Apply the “Form_Load” and “Button click event handlers” rules with Regex conditions to the following code:
using System;
using System.Windows.Forms;
namespace WinFormsApp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
}
private void button2_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
}
}
}
The result will look something like this:

Sorting Members by Type Name
In CodeRush v20.2.3, we’ve added the capability to sort members by their type name. For example, If you want to sort fields by visibility, member type name, and name, you can now apply the following rule:
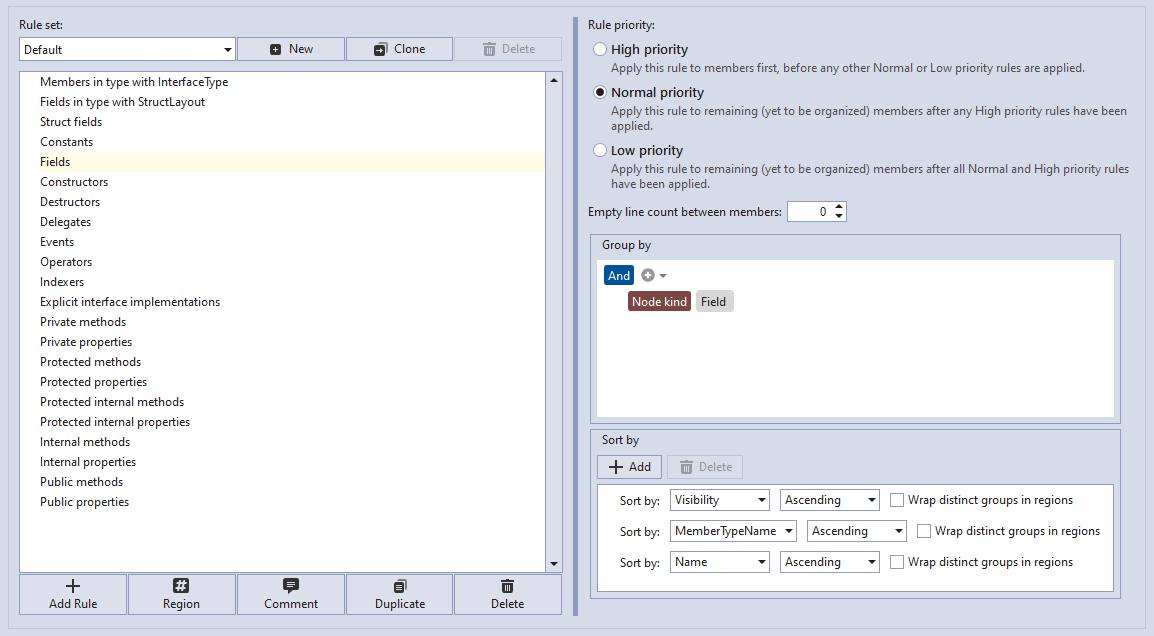
As an example, consider the following code:
namespace WinFormsApp
{
public class MyClass
{
public int prop4;
private string prop2;
private object prop3;
public byte prop1;
public int Prop4 {
get { return prop4; }
set { }
}
private string Prop2 {
get { return prop2; }
set { }
}
private object Prop3 {
get { return prop3; }
set { }
}
public byte Prop1 {
get { return prop1; }
set { }
}
public void MyMethod()
{
Prop1.ToString();
Prop2.Clone();
Prop3.ToString();
Prop4.ToString();
}
}
}
After running Organize Members you can see the following result:
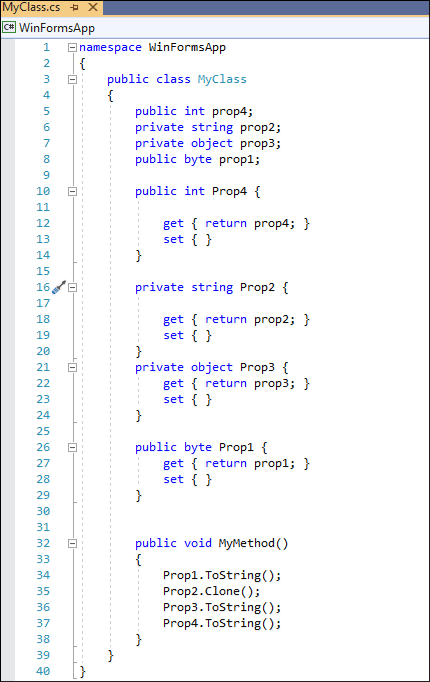
Conclusion
We hope the new Organize Members enhancements will help you automate grouping and sorting of your code and make the code more readable and easier to understand and maintain.
As always, we welcome your feedback. If you have specific questions or need assistance with CodeRush, feel free to let us know in the comments below or contact us through our Support Center (support@devexpress.com).
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.