In the next major release, v18.1, we've updated the client-side API format for all DevExpress Bootstrap ASP.NET Core controls. The changes in the API make it more intuitive and will improve your development experience.
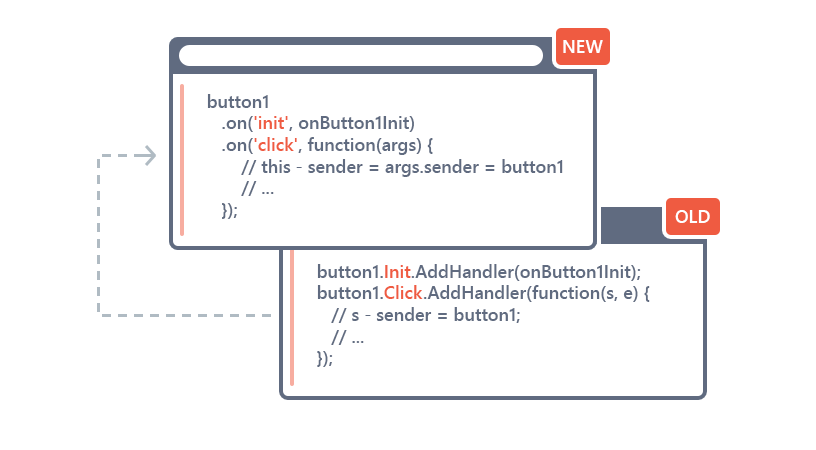
Let's see what is changed.
camelCase Methods

Camel case (stylized as camelCase ...) is the practice of writing compound words or phrases such that each word or abbreviation in the middle of the phrase begins with a capital letter, with no intervening spaces or punctuation. -Wikipedia
In JavaScript, it's common to use lower camel case for method names where the first letter is lowercase. Therefore, all methods that were previously written like this:
GetItem()
GetEnabled() / SetEnabled()
GetIconCssClass() / SetIconCssClass()
GetText() / SetText()
GetVisible() / SetVisible()
Starting with v18.1, they'll now use camelCase:
getItem()
setEnabled() / getEnabled()
setIconCssClass() / getIconCssClass()
setText() / getText()
setVisible() / getVisible()
Event Handling
There's big changes in event handling because with the v18.1 release, we're making it easier to work with event handlers.
For example, let's say that you want to handle the client-side Init
and Click
events of the DevExpress Bootstrap ASP.NET Core Button control. Then you would use the following JavaScript functions as handlers for the respective events:
function onButton1Init(s, e) {
// ...
}
function onButton1Click(s, e) {
// ...
}
This improves on the old way of declaring functions and then attaching the handlers for events:
button1.Init.AddHandler(onButton1Init);
button1.Click.AddHandler(function(s, e) {
// s - sender = button1;
// ...
});
button1.Click.AddHandler(onButton1Click);
New Functions: 'on', 'off', & 'once'
New for the v18.1 release, is the ability for every control to provide the on
function. The on
function accepts an event name and a handler function:
on = function (eventName, handler){
...
return this;
}
These new functions return the object they're called on, and so calls to them can be chained. This allows you to write fluent syntax to assign event handlers:
button1
.on('init', onButton1Init)
.on('click', function(args) {
// this - sender = args.sender = button1
// ...
})
.on('click', args => { // es6 or TS
// this - external context;
// args.sender = button1
// ...
})
.on('click', onButton1Click);
Detach with 'off'
There's also a corresponding off
function that allows you to detach handlers:
button1
.off('init', onButton1Init)
.off('click', onButton1Click);
button1
.off('init', onButton1Init)
.off('click'); // remove all handlers for the click event
button1
.off() // remove all event handlers
'once' Function
We also added the once
function that is identical to the on
function above, except that the handler will be removed after its first invocation.
JavaScript Promises
Also with the v18.1 release, we will provide overload functions that return JavaScript Promises for callback methods, e.g. BootstrapGridView.performCallback()
. So without promises, we would use this approach:
grid.performCallback('myParameter', function (result) {
// This callback function will be executed when the result comes from the server
// The 'result' argument is a value received from the server
console.log(result);
});
By using JavaScript Promises then we can write fluent code instead:
grid.performCallback('myParameter')
.then(function (result) {
// The 'result' argument is a value received from the server
console.log(result);
} );
If you use async/await
syntax (supported in ECMAScript 2016+), then the code can be simplified:
// The `result` argument is a value received from the server
let result = await grid.performCallback('myParameter');
console.log(result);
VS IntelliSense
Last, but not least, with the official 18.1 release, we will provide type definitions for the new client-side API so that you get IntelliSense in Visual Studio.
Old API Still Available
The old client-side API has been marked as deprecated in the v18.1 release (and beyond). However, it has not been removed from v18.1. I recommend using the new API in your projects, but there is no need to change the current applications immediately.
Rest assured that you'll be able to upgrade to v18.1 and get all these great changes without your previous client-side code breaking.
Try v18.1 CTP Today
You can access the v18.1 CTP of the DevExpress Bootstrap for ASP.NET Core via NuGet:
Use the https://nuget.devexpress.com/early-access/api NuGet feed to install the DevExpress.AspNetCore.Bootstrap package.
Or use the following dotnet CLI command to install the controls:
dotnet add package DevExpress.AspNetCore.Bootstrap -s https://nuget.devexpress.com/early-access/api -v 18.1.2-pre-18082
Learn more about the limited time v18.1 CTP here.
Feedback
Your feedback is valuable to us. For these new changes to the Client-Side API, we've created a support ticket where I'd love for you to give us your input: T620627
Thanks!
Email: mharry@devexpress.com
Twitter: @mehulharry
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.