As you may know, we expect to ship DevExpress Reports v23.1 in a couple of months. While you wait for the official release of our next major version, I thought it important to summarize a few DevExpress Reports-related features introduced in our v22.2 release cycle. Should you have any questions or require assistance with the features/capabilities described herein, please create a DevExpress Support Center ticket – we’ll be happy to follow-up.
Report Wizard — Custom Report Templates
When you or users don’t have time to create a report from scratch, look no further than the DevExpress Report Wizard.
In our v22.2 release cycle, we extended Report Wizard-related APIs so you can add predefined report templates to your solution. Our new Report Wizard API allows you to manage the report generation process once users complete the last step in the wizard (apply data source or report layout modifications). And yes, you can even add a custom page to the wizard when necessary.
In the following example, I’ll demonstrate the customization process step-by-step. You’ll see how to add a custom template, incorporate a custom page, and generate your custom report.
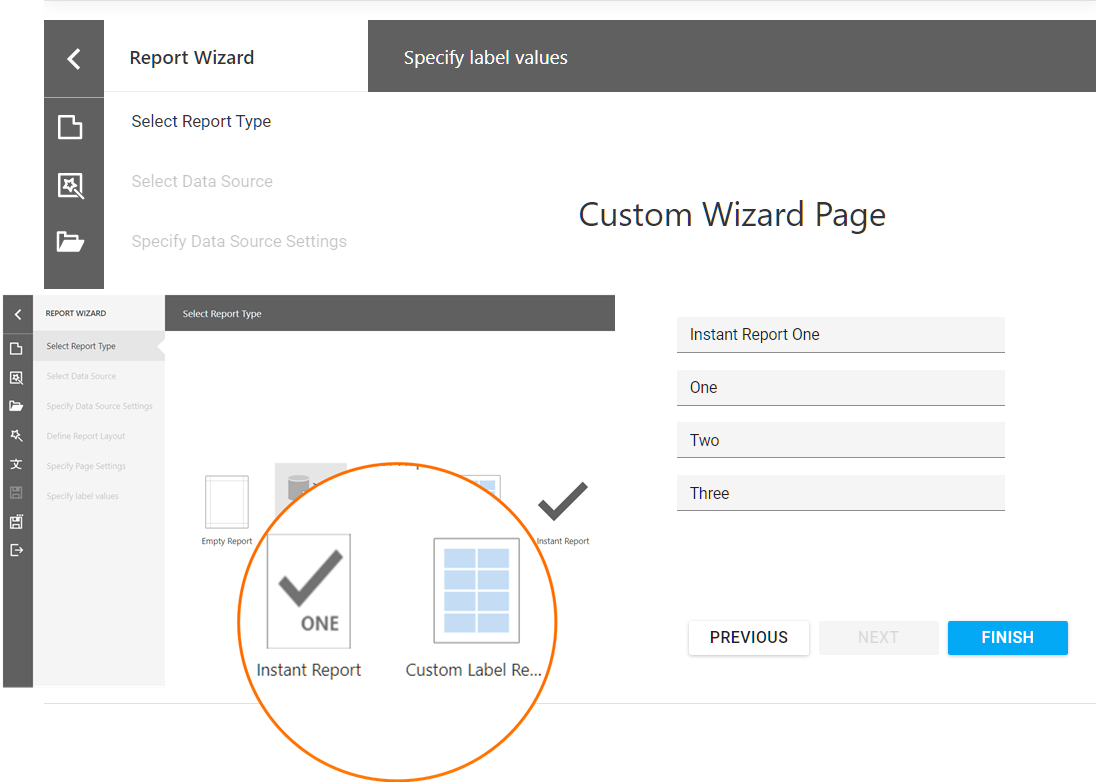
Implement and Register a Wizard Customization Service
The descendant of our ReportWizardCustomizationService offers a set of methods designed to register and process custom templates.
We’ll start with our CustomizeReportTypeList method implementation. This method obtains a collection of report templates, with built-in report elements. You can remove default (if unnecessary/unwanted) report templates and/or add custom templates as needed.
A custom template represents a ReportWizardTemplate data contract and allows you to specify:
- The template identifier
- The displayed text
- The template icon (you can use an SVG image template as a CSS Image class)
The Report Wizard uses the template collection passed to the CustomizeReportTypeList method to display report templates for the end-user:
public class InstantReportWizardCustomizationService :
ReportWizardCustomizationService {
public enum CustomReportType {
CustomLabelReport,
InstantReport
}
public override void CustomizeReportTypeList(
ReportWizardTemplateCollection predefinedTypes) {
predefinedTypes.Add(new ReportWizardTemplate() {
CanInstantlyFinish = true,
ID = nameof(CustomReportType.InstantReport),
Text = "Instant Report",
ImageTemplateName = "instant-report"
});
predefinedTypes.Add(new ReportWizardTemplate() {
CanInstantlyFinish = true,
ID = nameof(CustomReportType.InstantReport),
Text = "Instant Report",
ImageClassName = "instant-report-image"
});
predefinedTypes.Add(new ReportWizardTemplate() {
ID = nameof(CustomReportType.CustomLabelReport),
Text = "Custom Label Report",
ImageTemplateName = "dxrd-svg-wizard-LabelReport"
});
}
// ...
}

Once the user selects a template and completes the wizard, the Report Wizard calls the TryCreateCustomReport method. TryCreateCustomReport generates the report, modifies report settings, and returns an instance of the report to the Report Designer. The report template identifier allows you to determine which report instance to generate.
public class InstantReportWizardCustomizationService :
ReportWizardCustomizationService {
// ...
public override XtraReport TryCreateCustomReport(XtraReportModel model,
object dataSource, string dataMember, CustomWizardData customWizardData,
XtraReport report) {
if(Enum.TryParse(customWizardData.ReportTemplateID,
out CustomReportType customReportType)) {
if(customReportType == CustomReportType.InstantReport) {
return new InstantReport() {
Name = customWizardData.ReportTemplateID,
DisplayName = customWizardData.ReportTemplateID
};
}
}
return base.TryCreateCustomReport(model, dataSource,
dataMember, customWizardData, report);
}
// ...
}
Before the report is sent to the DevExpress Report Desiger, our CustomizeReportOnFinish method is called to make necessary/final adjustments.
public class InstantReportWizardCustomizationService :
ReportWizardCustomizationService {
// ...
public override void CustomizeReportOnFinish(XtraReport report) {
if (report.Bands.GetBandByType(typeof(ReportHeaderBand)) == null)
report.Bands.Add(new ReportHeaderBand() {
Controls = {
new XRLabel() {
Text = string.Format("Instant Report {0:MMM dd}",
System.DateTime.Today),
SizeF= new System.Drawing.SizeF(650F, 100F),
Font = new DevExpress.Drawing.DXFont("Arial", 24)
} }
}); ; ;
}
// ...
}
Add a Custom Wizard Page
To define a custom report page, you’ll need to add an HTML template. This custom report page can address a variety of usage scenarios. For instance, you can ask users to input additional data, add the data to a session object, and then use it via the CustomWizardData data contract in the TryCreateCustomReport method.
public class InstantReportWizardCustomizationService :
ReportWizardCustomizationService {
// ...
public override XtraReport TryCreateCustomReport(XtraReportModel model,
object dataSource, string dataMember, CustomWizardData customWizardData,
XtraReport report) {
if(Enum.TryParse(customWizardData.ReportTemplateID,
out CustomReportType customReportType)) {
if(customReportType == CustomReportType.InstantReport) {
return new InstantReport() {
Name = customWizardData.ReportTemplateID,
DisplayName = customWizardData.ReportTemplateID
};
} else if(customReportType == CustomReportType.CustomLabelReport) {
var labelReport = new LabelReport();
labelReport.ApplyCustomData(customWizardData.Data);
return labelReport;
}
}
return base.TryCreateCustomReport(model, dataSource, dataMember,
customWizardData, report);
}
// ...
}}
If you’re ready to customize the DevExpress Report Wizard, we’ve published a sample in the following GitHub repository: Web Reporting (ASP.NET MVC, ASP.NET Core and Angular) - How to Customize the DevExpress Report Wizard Customization and Hide Data Source Actions within our Report Designer.
This sample will show you how to manage report templates within the Report Wizard and use custom user input to modify the newly generated report.
Report Wizard — Data Source Management
As you may already know, we streamlined how users add and configure data sources within the DevExpress Report Designer. Our Report Designer’s Field List (v22.2) offers a number of advantages over its predecessors:

As the screenshot above illustrates, a new Add Data Source button allows you to invoke our Data Source Wizard. The wizard includes a list of available data sources (data sources stored inside a given report). Users can add a new data source to this report using data connections supplied to the wizard via related report services.
.
We also updated icons and added new data source action buttons. For instance, Rebuild Schema and Rename Data Source buttons are now available for each data source in the Field List. The following image summarizes the capabilities of individual action buttons.
:
Note: Our ReportDesignerDataSourceSettings class allows you to hide data source actions and the Add Data Source button itself. If you hide the Add Data Source action, the Add Data Source menu command will also be hidden.
Report Control Rendering
XRBarCode and XRGauge report controls now ship with enhanced design-time support (WYSYWIG rendering). When used in the DevExpress Report Designer, our Barcode control now renders a real barcode image (based on selected symbology and specified text data). In much the same way, our Gauge controls now renders a real gauge (based on selected gauge type and specified data property values) when displayed within the Report Designer.
v22.1 |
v22.2 |
 |
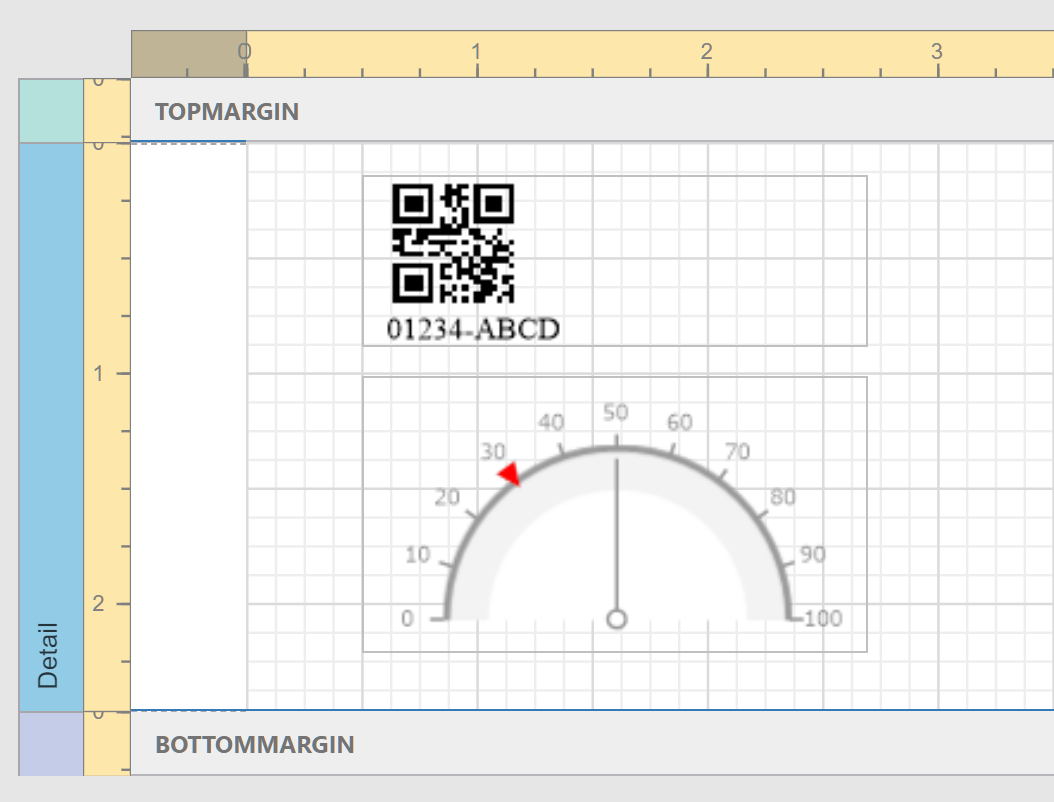 |
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.