I'm sure you are aware of Microsoft's recent advances for Visual Studio, .NET Core, and their new efforts in creating/deploying/using web applications. Along with these accomplishments -- and also to give our ASP.NET team something else to do! -- we are releasing a set of seven native components to help you build rich browser-based UI using Blazor Components.
Blazor? Razor Components?
Let's take a quick detour to understand what these technologies are, and how they could help you, and why we decided to create this new suite.
Firstly, though, let's quickly talk about developing client-side web apps. Traditionally, this meant you could only use JavaScript. In fact, you needed JavaScript to do anything on the client-side.
With Blazor / Razor Components you can now write rich interactive client-side web UI using .NET! This means you can write client-side web apps using C# and Razor syntax with .NET assemblies. In fact, the name comes from combining Browser + Razor = Blazor.
Let's take a look at how Blazor works.
Blazor - a client-side framework
This is a fairly new framework that Microsoft created with an open-source product called WebAssembly. WebAssembly? To quote Mozilla, WebAssembly...
...is a low-level assembly-like language with a compact binary format (Wasm) that runs with near-native performance and provides languages such as C/C++ and Rust with a compilation target so that they can run on the web. It is also designed to run alongside JavaScript, allowing both to work together.
What Microsoft did with Blazor is to run .NET code, using Mono compiled under WebAssembly, into Wasm code that could execute in the browser.
Microsoft's Blazor documentation has a good breakdown of what occurs when a Blazor app is built and run in a browser:
- C# code files and Razor files are compiled into .NET assemblies.
- The assemblies and the .NET runtime are downloaded to the browser.
- Blazor bootstraps the .NET runtime and configures the runtime to load the assemblies for the app. Document Object Model (DOM) manipulation and browser API calls are handled by the Blazor runtime via JavaScript interop.

Image by Daniel Roth, Microsoft Docs
Now you know how Blazor works in the browser, let's take a look at what Blazor is...
Blazor is a single-page app framework for building interactive client-side Web apps with .NET. Blazor uses open web standards without plugins or code transpilation. Blazor works in all modern web browsers, including mobile browsers. -Microsoft Docs
What benefits does this give you?
- You don't need to use JavaScript to create the UI for your single-page application (SPA). You can write everything in C# or VB.
- Blazor apps running on WebAssembly render client-side and you also have access to the .NET ecosystem.
- You can share the server-side and client-side logic. Full-stack development, in other words.
- Since it's all .NET, all the time, you can rely on its performance, reliability, and security.
- You're using Visual Studio 2019 for development.
It does have some downsides, though:
- Debugging is a pain. Remember: what's executing in the browser is Wasm code, not C#, not JavaScript.
- Download times: you get the runtime as WASM, but most of your app is downloaded as normal .NET assemblies
- Currently, Microsoft describe it as "experimental".
While Blazor on the client-side is still an experimental project, Microsoft has been shipping a steady stream of updates, so hopefully the experimental label will be gone soon. They're actively working to improve the downsides too and we look forward these updates.
Razor Components
Now a quick word about Razor Components:
A Razor Component is a piece of UI, such as a page, dialog, or data entry form. Components handle user events and define flexible UI rendering logic. Components can be nested and reused.
Components are .NET classes built into .NET assemblies that can be shared and distributed as NuGet packages. The class can either be written in the form of a Razor markup page (.cshtml) or as a C# class (.cs). -Microsoft Docs
If you've used Angular or React, then you're familiar with the concept of everything in the UI being a component. Razor Components behave the same way. This component model gives you more control over how you build and control your UI.
Razor Components are what you use to create Blazor apps. One key advantage of Razor Components is they can be used either on the client-side or server-side!
Server-side Blazor
The benefits of Blazor in the browser are significant, but problematic. So, to address the problems, Microsoft introduced what might be called "server-side Blazor". In short, the UI is hosted and rendered on the server and then sent to the client (and updated) via SignalR over a real-time connection, a web socket. In other words, all of the benefits I just mentioned, with none of the issues. All C# and Razor syntax, and no need for JavaScript.
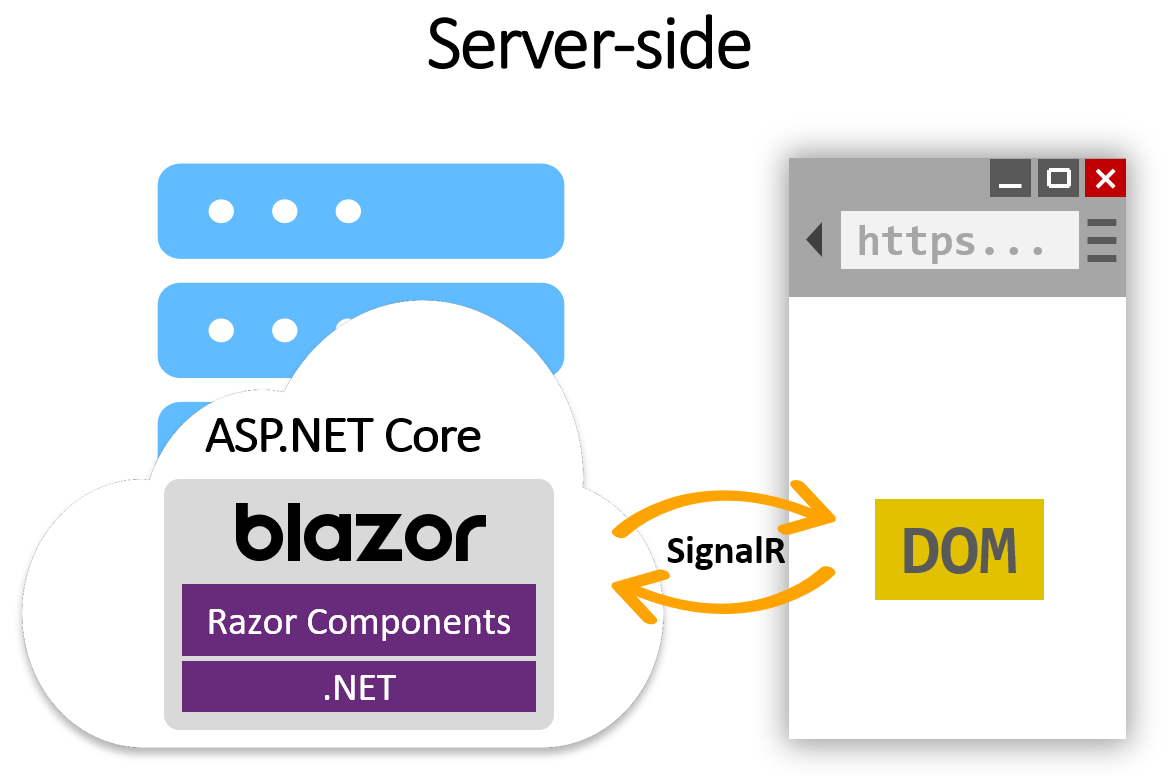
Image by Daniel Roth, Microsoft Docs
What benefits does this give you?
- Faster app startup
- Runs on .NET Core (fast, not limited by WebAssembly or browser sandbox)
- Simpler for some scenarios
It does have some downsides, though:
- Requires a connection (no offline)
- Latency
- Requires more server resources
However, Microsoft is actively updating Blazor on their GitHub repo and we look forward to see this new technology develops in the coming months.
DevExpress UI for Blazor / Razor Components
To celebrate this new technology, we have designed and created a new suite of Razor Components for Blazor. Best of all, these new components will work for both client and server side Blazor!
The controls are native for Blazor and newly created by our ASP.NET developers. The same devs who have built our excellent ASP.NET controls.
We are releasing them as a free "early access" preview through Nuget. There are seven components so far: a Grid (of course!), a Pivot Grid, and various editors (a Combo Box, a Spin Edit, a Date Edit, Text Box, and Pager) that can be used within the grid.
The grid is the most complete: it provides data binding, editing, paging, filtering, row selection, some pre-defined column types, and the use of templates.
In fact, the data binding supports component parameters, which bind a property values across components.
There are a couple of events for editing too:
namespace DevExpress.Blazor {
public class DxDataGrid {
[Parameter] internal RenderFragment ChildContent { get; set; }
[Parameter] IEnumerable<T> Data { get; set; }
[Parameter] protected bool ShowColumnHeaders { get; set; } = true;
[Parameter] protected bool ShowFilterRow { get; set; } = true;
[Parameter] protected bool ShowPager { get; set; } = true;
[Parameter] protected bool AllowRowSelection { get; set; } = true;
[Parameter] protected int PageSize { get; set; }
[Parameter] Action<T> SelectedItemChanged { get; set; }
[Parameter] T SelectedDataItem { get; set; }
[Parameter] Action<T> RowRemoving { get; set; }
[Parameter] Action<T, Dictionary<string, object>> RowUpdating { get; set; }
[Parameter] Action<Dictionary<string, object>> RowInserting { get; set; }
}
...
Obviously we have plans for more components and more functionality, and I'll talk about this in a moment.
Download the preview from NuGet
If you wish to test the DevExpress UI for Blazor / Razor Components, download the DevExpress.RazorComponents.nupkg
from the DevExpress NuGet Early Access feed:
https://nuget.devexpress.com/early-access/api
Once you've added this feed to Visual Studio 2019, you'll see the DevExpress.RazorComponents package:
This preview is made available under the DevExpress Preview end-user license. To gain access to the build free of charge, you will need a DevExpress.com account. You can either create a free account to test our NuGet package or you can get a free 30-day trial of all our controls.
Demo
Experience the DevExpress Razor Components online here:
https://demos.devexpress.com/razor-components/
Get Started
We've created a guide to help you learn how to create a new project and use DevExpress UI for Blazor / Razor Components:
https://github.com/DevExpress/RazorComponents/
Watch Training Videos
We've prepared four excellent getting-started videos to help you learn Blazor:
- Getting Started
- Component Structure
- Dependency Injection & Data Access
- JavaScript Interop
Plans
First, these preview components will be further developed with new features and functionality. Obviously, as Microsoft releases updates to .NET Core, so shall we.
Second, although we have many plans for more components within this new ecosystem, as with all of our future projects we rely on feedback from you, our customers, on what we should be looking at and considering. Send us emails, open up support tickets, let us know.
Along those lines, please take a moment to complete this short survey:
Thanks!
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.