There it was staring me in the face for the last couple of weeks:

That is indeed a TO-DO task that is slightly overdue. A little background:
Several weeks ago I encountered a thread explaining a feature request dealing primarily with exporting reports to PowerPoint. I said I would hack something together in the next couple of weeks. I finally decided to make it work!
Here is a standard report as previewed in Visual Studio:

This report is generated from the Northwind database that ships with our products. In it we have a chart per product category describing the units in stock aligned with units on order to get a feeling for how well a product is selling versus current warehouse levels (thanks to Bryan Wood for helping me out here!). I wanted to export this report to PowerPoint. The results are shown below:
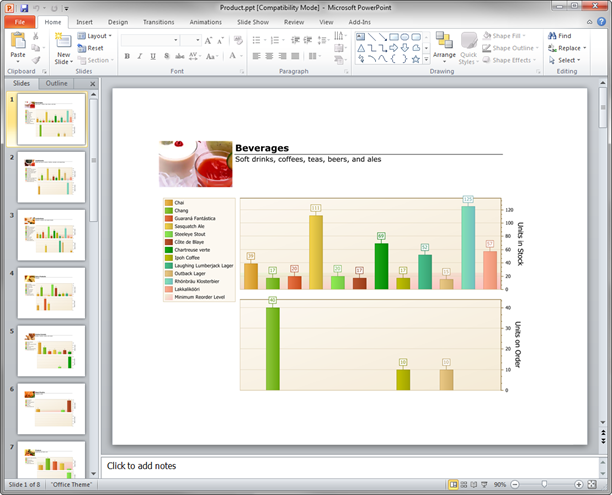
I thought it was pretty neat! Notice that each slide corresponds to a page in the report. The process was pretty simple:
- Create a mechanism for creating slides in a PowerPoint Presentation
- Export each page of the report to an image
- Create a slide for each page with the corresponding image inserted
- Save the File
- Done!
Some code:
Exporting to PowerPoint
1: public class Presentation
2: {
3: Alias.Application _powerPoint;
4: Alias.Presentation _presentation;
5:
6: ~Presentation()
7: {
8: _powerPoint = null;
9: _presentation = null;
10: GC.Collect();
11: GC.WaitForPendingFinalizers();
12: GC.Collect();
13: GC.WaitForPendingFinalizers();
14: }
15:
16: public Presentation()
17: {
18: _powerPoint = new Alias.Application();
19: _presentation = _powerPoint.Presentations.Add(
20: WithWindow: Microsoft.Office.Core.MsoTriState.msoFalse);
21: }
22:
23: private int _slideNo = 0;
24: public void AddPage(string fileName)
25: {
26: _slideNo++;
27: var slide = _presentation.Slides.Add(_slideNo,
28: Microsoft.Office.Interop.PowerPoint.PpSlideLayout.ppLayoutBlank);
29: slide.Shapes.AddPicture(fileName,
30: Microsoft.Office.Core.MsoTriState.msoFalse,
31: Microsoft.Office.Core.MsoTriState.msoTrue, 0, 0);
32: }
33:
34: public void SaveAs(string filename)
35: {
36: if (File.Exists(filename))
37: File.Delete(filename);
38:
39: _presentation.SaveAs(filename);
40: _presentation.Close();
41: _powerPoint.Quit();
42: }
43: }
The key here is to add a reference to the Microsoft PowerPoint 14.0 Object Library (a COM reference). This will allow you to create an instance of PowerPoint, add slides ad-hoc, and subsequently save the file.
Converting from Report to PowerPoint
1: private void ExportToPowerPoint()
2: {
3: // To the desktop (for demo purposes)
4: var desktop = Environment.GetFolderPath(
5: Environment.SpecialFolder.DesktopDirectory);
6: // image export options
7: ImageExportOptions exportOptions = new ImageExportOptions
8: {
9: ExportMode = ImageExportMode.SingleFilePageByPage,
10: Format = ImageFormat.Png,
11: PageBorderColor = Color.White,
12: Resolution = 600
13: };
14:
15: // PowerPoint Presentation
16: Presentation p = new Presentation();
17:
18: // go through each page
19: for (int i = 0; i < _report.Pages.Count; i++)
20: {
21: // export image
22: var file = string.Format(@"{0}\{1}.png", desktop, i);
23: exportOptions.PageRange = (i + 1).ToString();
24: _report.ExportToImage(file, exportOptions);
25: // add the image to the presentation
26: p.AddPage(file);
27: // clean up!
28: File.Delete(file);
29: }
30:
31: // save presentation to desktop
32: p.SaveAs(string.Format(@"{0}\Product.ppt", desktop));
33: }
Lines 21-28 do steps 2 and 3 while step 32 does the final step. Our fabulous report classes has a myriad of export options built right in. I leveraged the image export method to create the individual images. In the export options I set the actual export range (a single page) so that I could use each image as a slide in PowerPoint.
Now for some references:
S19976 - Export - Add a new export format - PPT (Power Point)
Q270590 - Export data to PPT
A Lesson I’ve Been Learning
I often get a lot of questions that go like this:
“Can you do X with your Reporting Suite?”
The answer is almost always –YES! The key is then understanding the reporting object model as well as the options available in this already rich reporting environment. My personal mandate is to make sure everyone gets to know the work of our brilliant reporting suite developers: specifically the ease and flexibility they baked into the system.
As always, if there are any comments and/or questions, feel free to get a hold of me!
Seth Juarez
Email: sethj@devexpress.com
Twitter: @SethJuarez
DXperience? What's That?
DXperience is the .NET developer's secret weapon. Get full access to a complete suite of professional components that let you instantly drop in new features, designer styles and fast performance for your applications. Try a fully-functional version of DXperience for free now: http://www.devexpress.com/Downloads/NET/
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.